Understanding Snake Order: A Comprehensive Guide
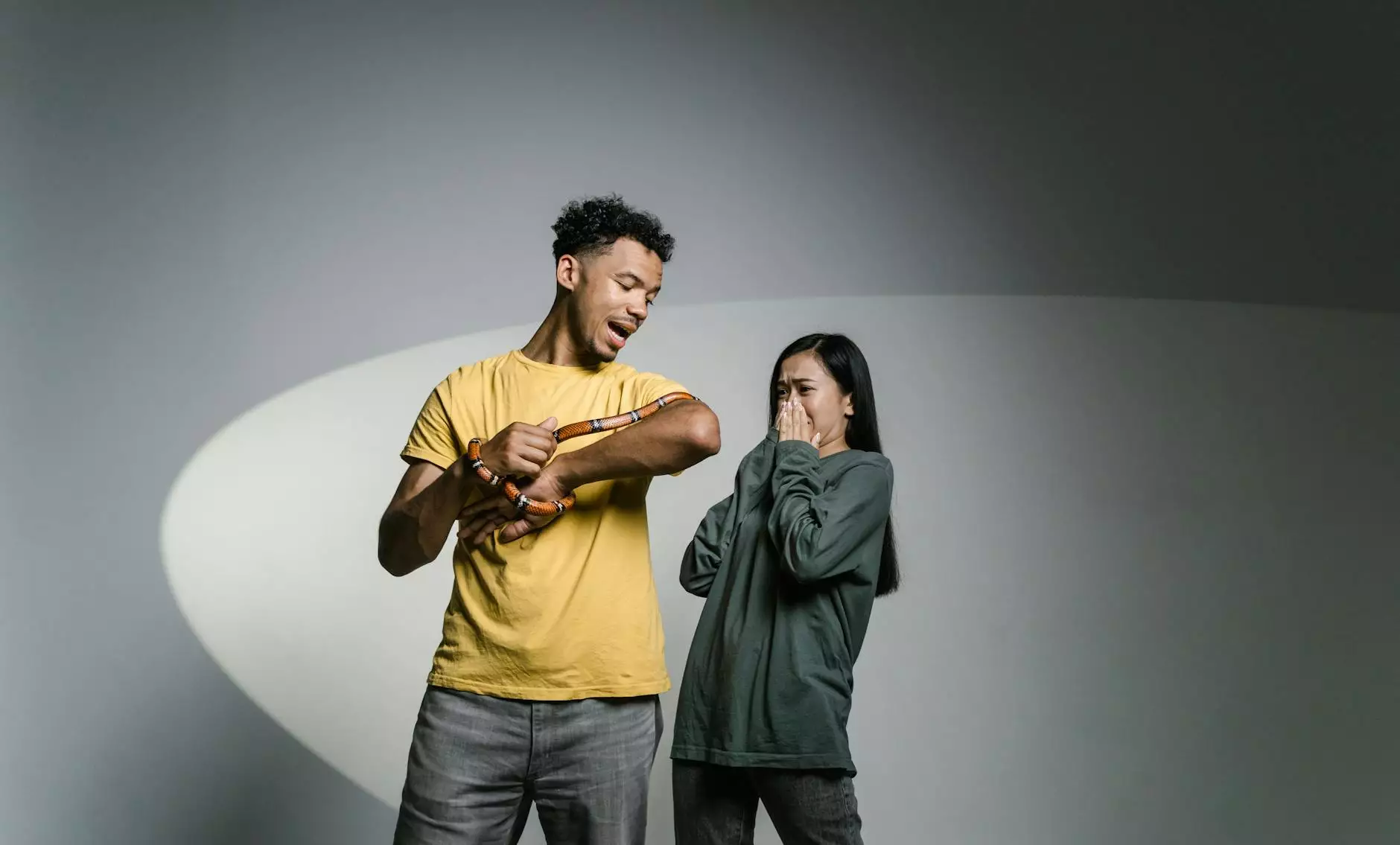
Snake order refers to a unique and fascinating way to traverse data structures, particularly two-dimensional arrays or matrices, in a zigzag pattern. This method not only aids in efficient data representation but also has intriguing applications in various fields, including programming and data science. In this detailed guide, we'll explore every aspect of snake order traversal, how it works, its benefits, and where you might encounter it, especially in the context of pet adoption, breeding, and reptile shops.
What is Snake Order Traversal?
Before delving into the intricacies of snake order, it's essential to understand the concept of matrix traversal. A matrix is a collection of numbers arranged in rows and columns, and the ability to navigate this data efficiently is crucial for tasks such as data analysis and programmatic data handling.
In a snake order traversal, the traversal direction alternates with each row. Let’s break down how this works:
- First row: Traverse from left to right.
- Second row: Traverse from right to left.
- Third row: Again, traverse from left to right.
- Fourth row: And so forth, alternating the direction for every new row.
This technique can be illustrated using a simple 3x3 matrix:
1 2 3 4 5 6 7 8 9The resulting sequence from a snake order traversal of this matrix would yield the output: 1, 2, 3, 6, 5, 4, 7, 8, 9. Such patterns not only make for interesting data sequences but also enhance the way we think about data structure traversal in programming.
Applications of Snake Order Traversal
Snake order traversal is more than just a theoretical concept; it has practical applications across various domains. Here are some notable examples:
1. Data Representation and Visualization
Understanding data in a visual format can transform how we interpret large datasets. Snake order is particularly useful when visualizing grids where alternating row patterns might yield clearer insights. For instance, in graphing pet adoption data on buyreptilesaus.com, using snake order can help present the data in a way that's both logical and aesthetically pleasing.
2. Game Development
In game development, particularly in grid-based games, snake order traversal can be used to determine how characters move or interact with the environment. This is critical for gameplay mechanics and can enhance the overall user experience.
3. Robotics and Pathfinding Algorithms
When programming robots, particularly those designed to navigate through a field or grid, snake order can help in optimizing their routes. This enables them to cover areas methodically without redundancy, which is essential when collecting data about surroundings, possibly relevant for businesses in pet shops that deal with environmental data.
Implementing Snake Order Traversal in Programming
Now that we've established the importance of snake order traversal, let’s take a closer look at how to implement it programmatically. Most programming languages can handle this concept, but let’s use Python for its simplicity and readability.
Python Implementation
def snake_order(matrix): if not matrix: return [] result = [] rows = len(matrix) cols = len(matrix[0]) for i in range(rows): if i % 2 == 0: # even row index for j in range(cols): result.append(matrix[i][j]) else: # odd row index for j in range(cols - 1, -1, -1): result.append(matrix[i][j]) return result # Example matrix matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] print(snake_order(matrix))This code will output: [1, 2, 3, 6, 5, 4, 7, 8, 9], showcasing the snake order traversal in action.
Why Understanding Snake Order is Beneficial for Businesses
For businesses in the pet industry, such as buyreptilesaus.com, understanding concepts like snake order traversal can enhance data handling and customer engagement. Here are some ways how:
1. Improved Data Handling
When running a business that involves numerous transactions, customer data, and inventory management, having a structured way to process this information ensures that you can derive valuable insights efficiently.
2. Better Customer Experience
With a clear understanding of how data is organized and retrieved, businesses can offer personalized services. For example, if your reptile shop uses data analytics to anticipate customer needs, you can ensure that the right snakes or other reptiles are available based on previous sales trends.
3. Competitive Advantage
In a competitive market, businesses that leverage data smartly will stand out. Incorporating advanced data traversal methods like snake order can facilitate better decision-making and operational efficiency.
Conclusion
In conclusion, the concept of snake order traversal provides an innovative way to approach data in a two-dimensional format. From programming to business applications, its relevance can’t be overstated. Whether you are a developer seeking to optimize your algorithms or a business owner looking to enhance your data strategy, the principles of snake order are invaluable.
As you consider the implications of this traversal technique, think about how you can integrate such strategies into your operations at buyreptilesaus.com. With the right application, the potential for growth and enhanced customer satisfaction is limitless.