How to Create a Chat App in Android
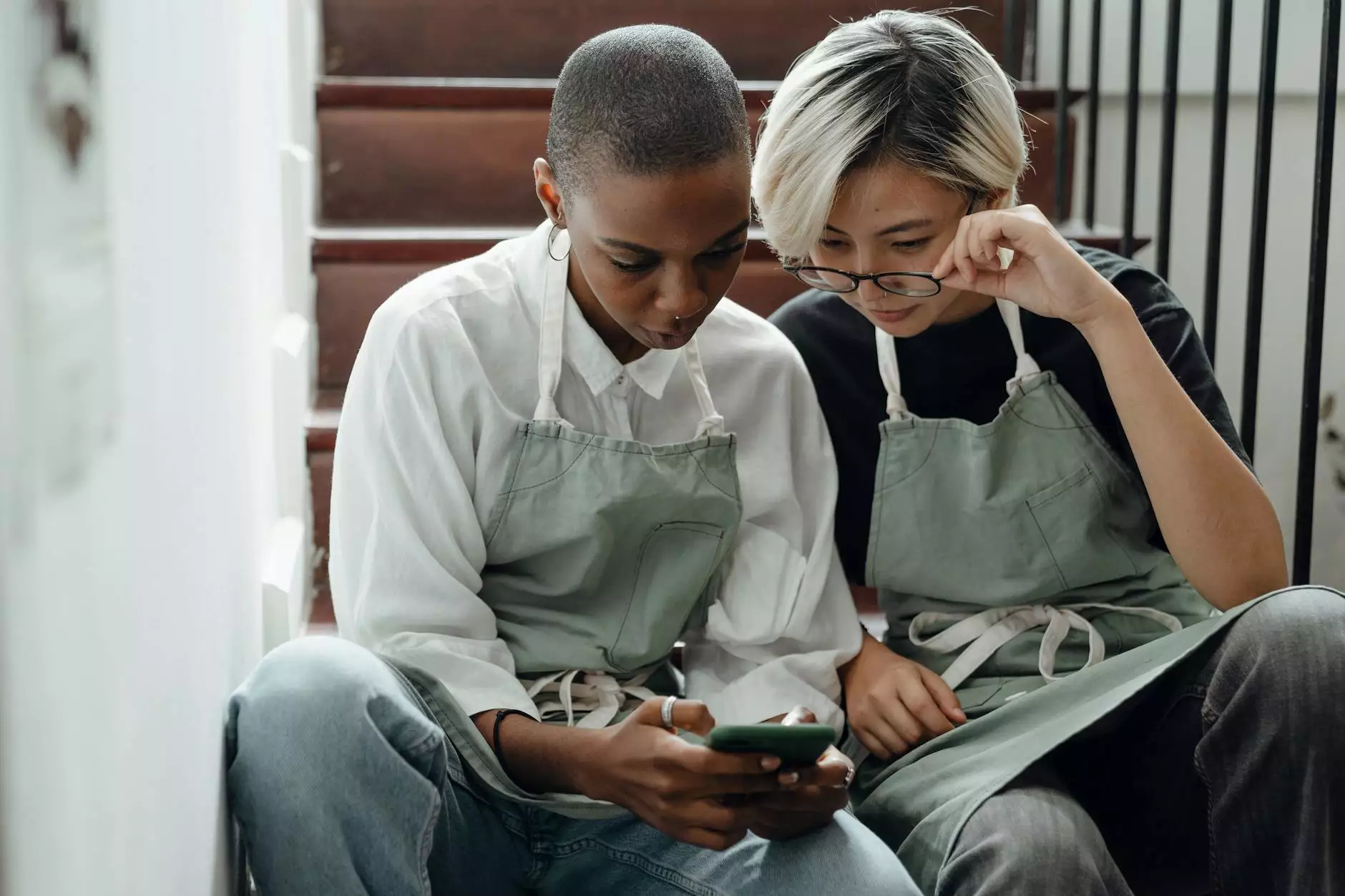
In today's digital age, communication is more crucial than ever. With millions of users interacting through various platforms, creating a chat app for Android can be a rewarding venture. This article will provide a comprehensive guide on how to create a chat app in Android, helping you to transform your innovative ideas into a functional application.
Understanding the Basics of a Chat Application
Before diving into the coding and designing process, it's essential to understand what constitutes a chat app. At its core, a chat application facilitates communication between users in real-time. The major components of a chat app include:
- User Authentication: Ensures that each user is verified before engaging in conversations.
- Real-Time Messaging: Utilizes technologies like WebSockets for seamless message delivery.
- User Interface (UI): The design of the chat window, buttons, and message display.
- Database: Stores user information and message histories securely.
- Notifications: Alerts users of new messages even when the application is not in use.
Step 1: Setting Up Your Development Environment
To start your journey in developing a chat app in Android, you need the right tools. Below are the key components you will need:
- Android Studio: The official Integrated Development Environment (IDE) for Android development.
- Java/Kotlin: Programming languages primarily used for Android applications.
- Firebase: A versatile platform that offers real-time database solutions, authentication services, and cloud messaging systems.
Step 2: Designing Your Application
The design aspect is crucial for user engagement and retention. Here are the primary elements to consider:
User Interface (UI) Design
Focus on creating a clean and intuitive UI. Use tools like Adobe XD or Figma to design your app layout. Key screens to consider include:
- Login/Register Screen: A simple interface for users to sign in or create an account.
- Chat Screen: The main feature where users send and receive messages.
- Profile Screen: Allows users to manage their personal information and settings.
User Experience (UX) Considerations
Ensure your app provides a smooth and enjoyable experience. This includes:
- Fast loading times
- Responsive alerts
- Easy navigation between different app sections
Step 3: Building the Backend
While the front end is important, the backend manages all data processing and storage. Here’s how to go about it:
Setting Up Firebase
Firebase provides comprehensive tools for building your chat app:
- Create a Firebase project in the Firebase Console.
- Enable Firebase Authentication, using methods such as email/password or Google Sign-In.
- Set up Firestore Database for storing messages and user data.
- Configure Cloud Messaging for push notifications.
Step 4: Implementing Real-Time Messaging
The core functionality of your chat app lies in real-time messaging. Using Firebase Firestore, you can achieve this with relative ease:
Sending Messages
To send messages, create a method that writes to the Firestore database. Each message should include the sender's ID, recipient ID, and the message content:
fun sendMessage(message: String, recipientId: String) { val messageData = hashMapOf( "senderId" to FirebaseAuth.getInstance().currentUser?.uid, "recipientId" to recipientId, "message" to message, "timestamp" to FieldValue.serverTimestamp() ) firestore.collection("messages").add(messageData) }Receiving Messages
To listen for new messages, implement a listener that triggers when new data is added to Firestore:
fun listenForMessages() { firestore.collection("messages") .whereEqualTo("recipientId", FirebaseAuth.getInstance().currentUser?.uid) .addSnapshotListener { snapshots, e -> if (e != null || snapshots == null) { return@addSnapshotListener } for (doc in snapshots.documentChanges) { if (doc.type == DocumentChange.Type.ADDED) { // Handle new message } } } }Step 5: User Authentication
For a chat app, ensuring the user's security is paramount. Firebase Authentication helps you manage this effectively:
Creating User Accounts
Utilize the following code to register a new user:
fun registerUser(email: String, password: String) { FirebaseAuth.getInstance().createUserWithEmailAndPassword(email, password) .addOnCompleteListener { task -> if (task.isSuccessful) { // Registration successful } else { // Handle failure } } }Logging In Users
Similarly, allow users to log in:
fun loginUser(email: String, password: String) { FirebaseAuth.getInstance().signInWithEmailAndPassword(email, password) .addOnCompleteListener { task -> if (task.isSuccessful) { // Login successful } else { // Handle failure } } }Step 6: Adding Push Notifications
Notifications enhance user engagement significantly. With Firebase Cloud Messaging (FCM), you can set up push notifications:
Configuring FCM
- Enable Cloud Messaging in the Firebase Console.
- Integrate the FCM SDK in your Android project.
- Write code to handle incoming messages and display notifications.
Step 7: Testing Your Application
After building your application, rigorous testing is essential to ensure everything functions smoothly:
Types of Testing
- Unit Testing: Test individual components to ensure they work as expected.
- Integration Testing: Test how different parts of the application work together.
- User Acceptance Testing: Gather feedback from real users to improve the app.
Step 8: Launching Your Chat App
Once testing is complete, it's time to release your app:
- Publish your app on the Google Play Store.
- Market your application through social media and other channels.
- Gather user feedback and make necessary updates and improvements.
Conclusion
Creating a chat app in Android is a challenging yet rewarding endeavor. By following the outlined steps and best practices, you can build a robust and user-friendly chat application that stands out in the crowded marketplace. Remember, continuous improvement and user feedback are key to the ongoing success of your application. This guide serves as a comprehensive roadmap for turning your idea into reality. Happy coding!